https://programmers.co.kr/learn/courses/30/lessons/42840
코딩테스트 연습 - 모의고사
수포자는 수학을 포기한 사람의 준말입니다. 수포자 삼인방은 모의고사에 수학 문제를 전부 찍으려 합니다. 수포자는 1번 문제부터 마지막 문제까지 다음과 같이 찍습니다. 1번 수포자가 찍는
programmers.co.kr
첫 번째 제출
더보기
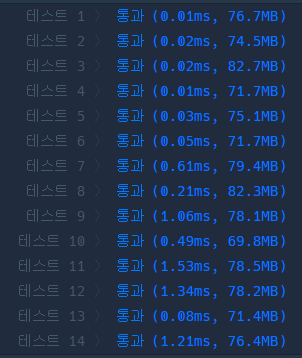
public static int[] solution(int[] answers) {
int[] answer = {};
int answersLength = answers.length;
int[] student1 = new int[answersLength], student2 = new int[answersLength], student3 = new int[answersLength];
//student1의 답
int stu1j = 0;
for(int i = 0; i < answersLength; i++) {
student1[i] = ++stu1j;
if( (stu1j) == 5) {
stu1j = 0;
}
}
//student2의 답
int stu2j = 2;
for(int i = 0; i < answersLength; i ++) {
if( (i % 2) == 0 ) {
student2[i] = 2;
} else if((i % 2) == 1 && (i % 8) == 1) {
student2[i] = 1;
} else if( (i % 2) == 1 ) {
if(stu2j == 5) {
stu2j = 2;
}
student2[i] = stu2j + 1;
stu2j++;
}
}
//student3의 답
int temp[] = {3, 3, 1, 1, 2, 2, 4, 4, 5, 5};
int stu3j = 0;
for(int i = 0; i < answersLength; i++) {
student3[i] = temp[stu3j++];
if(stu3j == 10) {
stu3j = 0;
}
}
//정답과 일치하는 경우 각각++
int stu1Count = 0, stu2Count = 0, stu3Count = 0;
for(int i = 0; i < answersLength; i++) {
if(answers[i] == student1[i]) {
stu1Count++;
}
if(answers[i] == student2[i]) {
stu2Count++;
}
if(answers[i] == student3[i]) {
stu3Count++;
}
}
//모든 경우 체크
if((stu1Count == stu2Count) && (stu2Count == stu3Count)) {
answer = new int[]{1, 2, 3};
} else if(stu1Count > stu2Count) {
if(stu1Count > stu3Count) {
answer = new int[]{1};
} else if(stu1Count == stu3Count) {
answer = new int[]{1, 3};
} else if(stu1Count < stu3Count) {
answer = new int[]{3};
}
} else if(stu2Count > stu1Count) {
if(stu2Count > stu3Count) {
answer = new int[]{2};
} else if(stu2Count == stu3Count) {
answer = new int[]{2, 3};
} else if(stu2Count < stu3Count) {
answer = new int[]{3};
}
} else if(stu1Count == stu2Count) {
answer = new int[]{1, 2};
}
return answer;
}
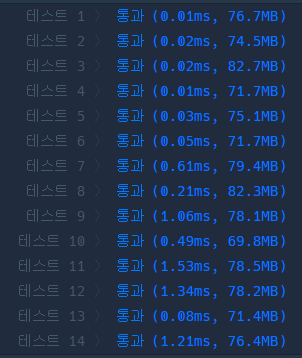
단순 Brute-Force로
나는 수포자 123의 답도 규칙 찾아서 하면 좋겠거니 하고 하나하나 규칙 찾아가며 갑 주입시켰는데
제출된 정답들 보니 그냥 예시에 있던 거 그대로 쓰고 그걸 활용했더라고
어떻게 저렇게 생각하지?
두 번째 제출
더보기
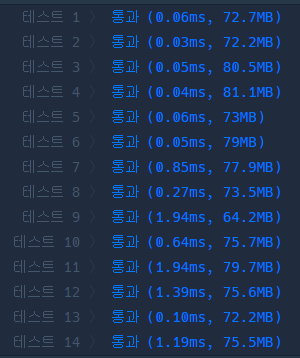
첫 번째 제출 시 모든 경우를 체크하는 부분이 진짜 마음에 안 들었지만 완전탐색이고 단순 Brute-Force로 하면 for와 if의 향연이 맞을 것이다 하고 일단 제출했었는데, 훨씬 깔끔하게 구현된 코드가 있길래 적용해봄
첫 번째 제출 코드에서 Math.max 써보려다가 어떻게 1 2 3을 구분해야할지 모르겠어서 안 썼는데 이렇게 하면 되는 거였음
public static int[] solution(int[] answers) {
//위와 동일
...
//
int[] count = new int[3];
for(int i = 0; i < answersLength; i++) {
if(answers[i] == student1[i]) {
count[0]++;
}
if(answers[i] == student2[i]) {
count[1]++;
}
if(answers[i] == student3[i]) {
count[2]++;
}
}
List<Integer> list = new ArrayList<>();
int maxCount = Math.max(count[0], Math.max(count[1], count[2]));
if(maxCount == count[0]) {
list.add(1);
}
if(maxCount == count[1]) {
list.add(2);
}
if(maxCount == count[2]) {
list.add(3);
}
answer = new int[list.size()];
for(int i = 0; i < list.size(); i++) {
answer[i] = list.get(i);
}
return answer;
}
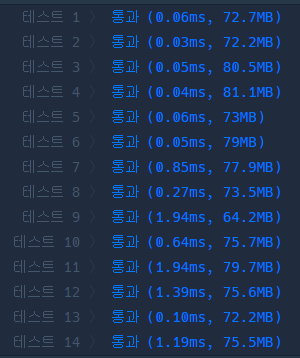
최종 제출
학생들 답도 효율적으로 넣고 싶어서 다시 수정
import java.util.ArrayList;
import java.util.List;
class Solution {
public static int[] solution(int[] answers) {
int[] answer = {};
int[] a = {1, 2, 3, 4, 5};
int[] b = {2, 1, 2, 3, 2, 4, 2, 5};
int[] c = {3, 3, 1, 1, 2, 2, 4, 4, 5, 5};
int[] count = new int[3];
for(int i = 0; i < answers.length; i++) {
if(answers[i] == a[i%5]) {
count[0]++;
}
if(answers[i] == b[i%8]) {
count[1]++;
}
if(answers[i] == c[i%10]) {
count[2]++;
}
}
List<Integer> list = new ArrayList<>();
int maxCount = Math.max(count[0], Math.max(count[1], count[2]));
if(maxCount == count[0]) list.add(1);
if(maxCount == count[1]) list.add(2);
if(maxCount == count[2]) list.add(3);
answer = new int[list.size()];
for(int i = 0; i < list.size(); i++) {
answer[i] = list.get(i);
}
return answer;
}
}